Cycles | JavaScript Camp
When an action needs to be repeated a large number of times, cycles are used in programming. For example, you need to display the text 📜 «Hello, World!» 🌎. Instead of repeating the same text output command 📜 two hundred times, a loop is often created that repeats 200 times what is written 🖊️ in the body of the loop. Each repetition is called an iteration.
Iteration in programming — in the broadest sense — the organization of data processing in which actions are repeated many times, without leading to calls 📞 themselves (as opposed to recursion). In a narrow sense, it is one step in an iterative, cyclical process.
A loop in JavaScript (and in all other languages👅) is a block of code📟 that repeats itself as long as a known condition is true. There are many different types of loops, but they all essentially do the same thing: they repeat an action several times.
The while() loop
The while
operator creates a loop that executes the specified instruction while the condition being tested is true. The logical value of the condition is calculated вычис before executing the loop body.
Syntax
while (condition) {
instruction // algorithm code - instructions
}
condition
is an expression whose boolean value is checked each time before entering the loop. If the value is true — true
✅, then the instruction is executed. When the value becomes false — false
❎, we exit the loop.
An instruction is an algorithm code that is executed every time the condition is true. To execute multiple instructions in a loop, use the {...}
block operator to group them. When using 1 command in the body of the loop, curly braces can be omitted.
Here is a simple example of a loop while (condition) {...}
function learnJavaScript() { let count = 0 // count is a counter let result = ‘Account:’ // change account to any while (count < 10) { result += count + ‘, ‘ count++ } return result }
Loading. ..
The count
in the example is 0
. The condition of our loop (it is written скоб in brackets) is that the block with the code will repeat itself over and over until (that is, the actual while
) count
is less than 10
.
Since the initial value of 0
is less than 10
, the code📟 runs. Each time the interpreter re-checks the ✔️ condition, if the condition is still true, then the code📟 will be run again. Each time we increment the counter value by 1
. Otherwise, the value would remain at 0
, so our condition counter <10
would always remain true, and our code📟 would loop forever!
As a result, after 10
cycles it will become 10
. After that, the interpreter will terminate the loop since the condition is false
❎ and go to the final lines of our code📟.
The for() loop
The for
expression creates a loop of three 3 optional expressions in parentheses, separated by semicolons.
Syntax
for ([initialization]; [condition]; [final expression])
{ expression }
initialization
— expression or definition of variables. This expression can optionally declare new variables using the let
keyword. These variables are visible only in the for
loop, i.e. in the same scope (for security).
condition
is an expression that is executed at each iteration of the loop. If the expression is true, the loop is executed. The condition is optional. If not, the condition is always considered true. If the expression is false, execution of for
is terminated.
final expression
— an expression that is executed at the end of the loop iteration. Occurs until the next condition is met. Usually used to
, decrement -
or update i + = 5
of a counter variable 🔔.
expression
— executable code of the algorithm while the condition of the loop is true
. To execute multiple expressions in a loop, use the
{...}
block to group those expressions. To avoid executing any expression in a loop, use an empty for (;;;)
expression.
Let’s calculate the sum of numbers from 0 to 100 👇:
function learnJavaScript() { let sum = 0 for (let i = 0; i <= 100; i++) { sum += i } return sum }
Loading…
Remember when we wrote our 1️⃣ first while()
what happened to our counter? We found that it is very important that it constantly changes (increment ++
helped us with this). Because if you forget about it, then the code📟 will fall into an infinite loop of loops
.
Well, situations like this happen quite regularly with while-loops
, which is why for
was done with a built-in counter!
Example for loop
When you first see the syntax📖 of a loop for
, you might think 🤔 that this is something very strange. But you should still study it 🧑🎓, since you will meet
like this many times:
function learnJavaScript() { let result = ‘Score: ‘ for (let i = 0; i < 10; i++) { result += i + ‘ ‘ } return result }
Loading…
Well, did you know? They should have! After all, this is practically the same code📟 that we used for the while
loop at the beginning of the chapter! The three parts of the cycle are separated by semicolons; they were all in that while loop, but in different places. Let’s take a closer look:
- First, we declare a counter variable —
let i = 0
. It is in the function itself and outside this loop that thisi
will be absent, and this is safe! - Next, we set a condition that the interpreter will check before each iteration of the loop (to determine whether it is worth starting the code at all).
An iteration is called one iteration of the loop (for example, if we had 10 startup cycles, then we can say that there were 10 code iterations).
- The third part of our code is
increment ++
(ordecrement --
). It runs at the end of each iteration to change our variable every time 🔔.
Conclusion
Almost always, when you know the number of iterations needed, you would rather work with for than with while. This is why for
loops are so popular. There are other cycles, but they are not so popular and if you want you can get acquainted with them here.
Problems?
Write to Discord chat.
Questions:
What is the name of a block of code that repeats itself over and over again until a given condition is no longer true?
- Cycle
- Condition
- Initialization
Which of the two loop operators is more popular?
for
while
break
How many messages will the following code output to the console?
let k = 0
while (k < 7) {
console.log('one more line!')
}
7
8
infinity
What is the character used to separate the parts of the for loop that are in parentheses?
&&
;
=!
If _______
always remains true, then the code can get stuck in an infinite _______
.
- condition / loop
- cycle / condition
What is the middle _____
of the three bracketed parts of a for
loop called?
- Expression
- Condition
- Increment
How many messages will the following code output to the console?
const maxNumer = 17
let n = 0
while (n <= maxNumer) {
console.log("Let's count!" + n)
n = n + 2
}
8
9
10
What is the term for a single (step) passage of the cycle?
- Iteration
- Interrupt
- Looping
In order to understand how much you learned this lesson, take the test in the mobile application of our school on this topic or in our telegram bot.
- MDN web doc. The article «The do … while loop»
- MDN web doc. For Loop Article
- MDN web doc. The while loop
- Iteration article, Javascript Express site
- While and for Loops
- Code for Teens: The Perfect Beginner’s Guide to Programming, Volume 1: Javascript — Jeremy Moritz
Contributors ✨
Thanks goes to these wonderful people (emoji key):
Dmitriy K. 📖 | Dmitriy Vasilev 💵 | Resoner2005 🐛 🎨 🖋 | Navernoss 🖋 🐛 🎨 |
Cycle.js — Видеоуроки | CourseHunter
Cycle.js — Видеоуроки | CourseHunter📌 Очень жаль, что всем известный персонаж решил начать войну. Мы молимся за Украину и за мир!
Cycle.js Fundamentals
itvdn
Загрузить
Пиши функциональный код быстро и чисто с помощью cycle, что позволит лучше структуризировать код на больших проектах с большой коммандой.
Этот материал находится в платной подписке. Оформи премиум подписку и смотри Cycle.js Fundamentals , а также все другие курсы, прямо сейчас!
Премиум
Урок 1. 00:03:30
The Cycle.js principle: separating logic from effects
Урок 2. 00:02:10
Main function and effects functions
Урок 3.
Customizing effects from the main function00:02:05
Урок 4. 00:04:06
Introducing run() and driver functions
Урок 5. 00:06:00
Read effects from the DOM: click events
Урок 6. 00:04:23
Урок 7.
00:05:22
Making our toy DOM Driver more flexible
Урок 8. 00:02:58
Fine-grained control over the DOM Source
- Урок 9.
00:02:53
Hyperscript as our alternative to template languages
Урок 10. 00:04:52
From toy DOM Driver to real DOM Driver
Урок 11.
00:04:44
Hello World in Cycle.js
Урок 12. 00:08:08
An interactive counter in Cycle.js
Урок 13. 00:12:19
Using the HTTP Driver
Урок 14. 00:06:40
Body-Mass Index calculator built in Cycle.
js
Урок 15. 00:03:17
Model-View-Intent pattern for separation of concerns
Урок 16. 00:08:05
Our first component: a labeled slider
Урок 17. 00:01:57
Using the component in the main() function
Урок 18.
00:04:31
Multiple independent instances of a component
Урок 19. 00:03:39
Isolating component instances
Урок 20. 00:03:30
Exporting values from components through sinks
Урок 21. 00:02:28
Overview of Cycle.
js
Комментарии
Только зарегистрированные пользователи могут комментировать️
Похожие
React JS
ReactJS
Марк Цукерберг настолько Марк Цукербер, что выкатил такое вот чудо в виде React Js. А ты дальше делаешь стартап на вордпрессе. Пробуй, учись, hello world запили. Марк Цукерберг. PS. На курс поставили скилл рак, но посмотрев бегло поняли что нихрена это не для раков, орк а то и дабл орк скилл.
Удален по просьбе правообладателя
Посмотреть
Express js
Express js
Express Быстрый, гибкий, минималистичный веб-фреймворк для приложений Node.js. Смотрим, юзаем, разбираемся…
Премиум
Посмотреть
net/course/hapi-js-node-angel-skiy»>hapi jshapi js / node
Богатый фреймворк для создания приложений
Hapi позволяет разработчикам сосредоточиться на написании многоразовой прикладной логики вместо того, чтобы тратить время на создание инфраструктуры.
Премиум
Посмотреть
Node JS
Node JS
Node js поможет тебе быстро и легко развернуть сервер со всеми плюшками которые тебе нужно. Это экономит кучу времени и нервов.
Премиум
Посмотреть
Angular 2
Angular 2
Выучил первый ангуляр ? Круто, забудь! Этот вообще не совместим с первым. Но Второй ангуляр проще, легче, и практически быстрей…
Удален по просьбе правообладателя
Посмотреть
Представляем Cycle.
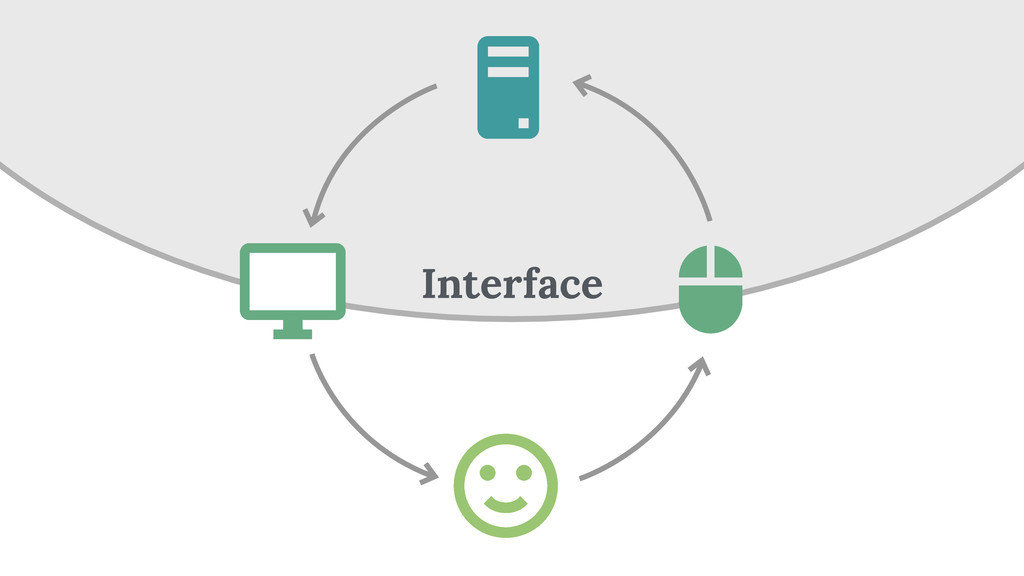
3 минуты чтения
Cycle.js — это функциональная и реактивная среда JavaScript для написания предсказуемого кода. Приложения, созданные с помощью Cycle.js, состоят из чистых функций, что означает, что они только принимают входные данные и генерируют предсказуемые выходные данные, не выполняя никаких эффектов ввода-вывода.
Какова основная концепция Cycle.js?
Cycle.js рассматривает ваше приложение как чистую функцию main() . Он принимает входные данные, которые представляют собой эффекты чтения (источники) из внешнего мира, и выдает выходные данные, являющиеся эффектами записи (приемниками), чтобы воздействовать на внешний мир. Драйверы, такие как плагины, которые обрабатывают эффекты DOM, эффекты HTTP и т. д., отвечают за управление этими эффектами ввода-вывода во внешнем мире.
Источник: Cycle.js
Функция main() построена с использованием примитивов реактивного программирования, которые максимизируют разделение задач и обеспечивают полностью декларативный способ организации кода. Поток данных в вашем приложении четко виден в коде, что делает его удобочитаемым и отслеживаемым.
Вот некоторые из его свойств:
Функциональный и реактивный
Поскольку Cycle.js является функциональным и реактивным, он позволяет разработчикам писать предсказуемый и разделенный код. Его строительными блоками являются реактивные потоки из таких библиотек, как RxJS, xstream или Most.js. Это значительно упрощает код, связанный с событиями, асинхронностью и ошибками. Эта структура приложения также разделяет проблемы, поскольку все динамические обновления части данных находятся в одном месте и не могут быть изменены извне.
Простой и лаконичный
Этот фреймворк очень прост в освоении и использовании, так как в нем очень мало концепций. Его основной API имеет только одну функцию, run(app, drivers). Кроме того, у нас есть потоки, функции, драйверы и вспомогательная функция для изоляции компонентов с областью действия. Большинство его строительных блоков — это просто функции JavaScript. Функциональные реактивные потоки могут создавать сложные потоки данных с очень небольшим количеством операций, что делает приложения в Cycle.js очень маленькими и удобочитаемыми.
Расширяемый и тестируемый
В Cycle.js драйверы — это простые функции, которые принимают сообщения от приемников и вызывают императивные функции. Все эффекты ввода-вывода выполняются драйверами, что означает, что ваше приложение — это просто чистая функция. Это упрощает замену драйверов. В настоящее время есть драйверы для React Native, HTML5 Notification, Socket.io и так далее. Кроме того, с Cycle.js тестирование — это просто вопрос подачи входных данных и проверки вывода.
Составной
Как упоминалось ранее, приложение Cycle.js, каким бы сложным оно ни было, представляет собой функцию, которую можно повторно использовать в более крупном приложении Cycle.js. Источники и приемники в этих приложениях действуют как интерфейсы между приложением и драйверами, но они также являются интерфейсом между дочерним компонентом и его родителем. Его компоненты — это не просто виджеты GUI, как в других фреймворках. Вы можете создавать компоненты веб-аудио, компоненты сетевых запросов и другие, поскольку интерфейс источников/приемников не является исключительным для DOM.
Подробнее о Cycle.js можно узнать на официальном сайте.
Читать далее
Представляем Howler.js, аудиобиблиотеку Javascript с полной кросс-браузерной поддержкой
npm на Node+JS Interactive 2018: npm 6, взлеты и падения фреймворков JavaScript и многое другое
InfernoJS v6.0.0 , похожая на React библиотека для создания высокопроизводительных пользовательских интерфейсов, вышла
Обязательно к прочтению в веб-разработке
Интервью
Cycle.js — Open Collective
Пожертвовать
Стать финансовым спонсором.
900″> Финансовые пожертвованияПериодические пожертвования
Покровители
Поддержите нас ежемесячным пожертвованием и помогите нам продолжать нашу деятельность.
Начиная с 2 долларов США в месяц
Последняя активность
Регулярный взнос
Серебряные спонсоры
Станьте Серебряным спонсором и разместите свой логотип в нашем README на Github.
250 долларов США в месяц
Последняя активность
Регулярный взнос
Золотые спонсоры
Станьте золотым спонсором и получите свой логотип на нашем сайте cycle. js.org (17000 uv/m) со ссылкой на ваш сайт и в нашем README на Github.
800 долларов США в месяц
Последняя активность от
Будьте первым, кто внесет свой вклад!
Индивидуальный взнос
Пожертвование
Сделайте индивидуальный разовый или повторяющийся взнос.
Последняя активность
Ведущие финансовые вкладчики
Организации
Verizon
9600 долларов США с апреля 2017 года
RELEX Solutions Май
90501 1,2 доллара США с 1,2HERP, Inc.
4px»> 1000 долларов США с июля 2019 годаFloat Left, LLC
500 долларов США с января 2018 г.
Piktochart
300 долларов США с ноября 2016 г.0003
198 долл. США с ноября 2018 г.
Hosted.nl
110 долл. США с ноября 2018 г.
HeadSpin
65 долл. США с марта 2022 г.
Физические лица
Арон Аллен
3500 долларов США
晃 羽田
1725 долларов США с октября 2016 года
Андре Стальц
4px»> 725 долларов США с сентября 2016 годаWayne Maurer 20016
октября
долларов США с октября 2016 года 3
Ронг Шен
450 долл. США с октября 2016 г.
Райан Хелмоски
350 долл. США с декабря 2017 г.
Натан
315 долл. США с апреля 2017 г.
Olavi Haapala
250 долларов США с июля 2017 г.
Оскар Тюнебинг
220 долларов США с октября 2016 года
Cycle.js — это все мы
4px»> Наши участники 116Спасибо за поддержку Cycle.js.
Андре Стальц
Admin
725 долларов США
Ник Джонстон
Ян ван Брюгге
Verizon
9 600 долларов США
Арон Аллен
60 5020 долларов США 9,09 Серебряные спонсоры3 4px»> 晃 羽田Сторонники
1725 долларов США
RELEX Solutions
Серебряные спонсоры
1250 долларов США
Мы ценим все усилия техподдержки…
HERP, Inc.
1000 долларов США
ンド開発で利用しているCycle.jsに資金援助をしました
Серебряные спонсоры
500 долларов США
Уэйн Морер
Сторонники
470 долларов США
Ронг Шен 3 Сторонники
03 06 450 долларов США
Райан Хелмоски
Сторонники
350 долларов США
Рабочий достоин своей зарплаты
Прозрачные и открытые финансы.
Ежемесячный финансовый взнос в Cycle.js (спонсоры)
Кредит от HeadSpin для Cycle.js •
+$5.00USD
Завершено
Взнос #528277
Ежемесячный финансовый взнос в Cycle.js (спонсоры)
Кредит от Geraud Henrion 09,24 апреля для Cyclenrion 023
+$2,00USD
Завершено
Взнос #497142
Ежемесячный финансовый взнос в Cycle.js (спонсоры)
Кредит от Reach Digital для Cycle.js •
3 + 20,00 долларов США0003
Завершено
Взнос #67147
$
Остаток на сегодня 3
700″ letter-spacing=»-0.4px»> 19 009,03 долл. СШАПредполагаемый годовой бюджет
398,02 долл. США
Connect
Давайте начнем!
Новости от Cycle.js
Обновления о нашей деятельности и прогрессе.
Обновление два раза в год
Привет, сторонники! Это реальное электронное письмо от Андре и Яна, просто для того, чтобы сообщить вам, какой прогресс был достигнут за последние 6 месяцев в ядре Cycle.js. Спасибо за вашу постоянную поддержку! Со времени последнего информационного бюллетеня в августе 2018 года, вот что произошло…
Подробнее
Опубликовано 4 января 2019 г. , автор Andre Staltz
Функциональная и реактивная среда интерфейса JavaScript, основанная на реактивных потоках, виртуальной модели DOM и расширяемости.
Поддерживает:
- Рендеринг Virtual DOM
- RxJS, большинство.js, xstream
- Машинопись
- Функциональные архитектуры
- Пользовательский отладчик Chrome DevTools
- Рендеринг на стороне сервера
- JSX
- React DOM и React Native
- Путешествие во времени
- Маршрутизация с помощью History API
Cycle.js — это фреймворк, в котором очень мало концепций для изучения. Основной API имеет только одну функцию: запустить (приложение, драйверы). Кроме того, есть потоки, функции, драйверы (плагины для различных типов побочных эффектов) и вспомогательная функция для изоляции компонентов с областью видимости.